How to work with Lists in Python
Lists are collection of items. The below are the characters of a Python list
- List stores the data in a sequence
- List is mutable (You can change/alter the items in a list)
- List is ordered collection (Maintains the insertion order)
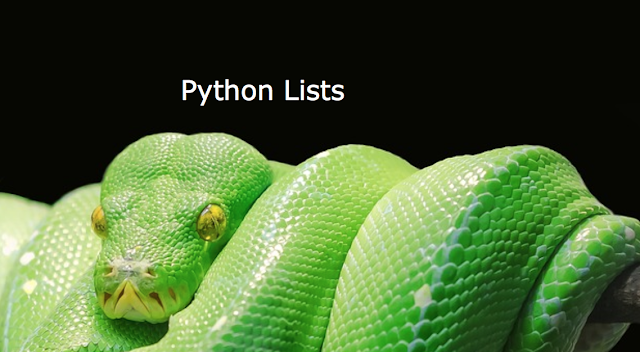
How to create a list in Python
Lets see different ways of creating a list in Python
An empty list can be created using [], the below example created an empty list
example_list = []
example_list = []
Creating a list with initial values,
example_list = [1, 2, 3, 4.5, 'a', 'b', [1, 2], (1, 2, 3), {1, 2}]
The above example describes that, list can have different type of data like integer, float, string, list, tuple, set, dictionary.
example_list = [1, 2, 3, 4.5, 'a', 'b', [1, 2], (1, 2, 3), {1, 2}]
The above example describes that, list can have different type of data like integer, float, string, list, tuple, set, dictionary.
How to access items from Python Lists
Items of a list can be accessed using indexation. Lists use 0 as beginning index. In Python lists can also be indexed in reverse order which starts from -1 so on to -n. Lets see some examples,
example_list = [1, 2, 3, 4, [1, 2]]
print(example_list[3])
print(example_list[-1])
output :
4
[1, 2]
print(example_list[3])
print(example_list[-1])
output :
4
[1, 2]
How to add items to a Python list
Append
example_list = []
example_list.append(1)
print(example_list)
example_list.append([2, 3])
print(example_list)
output :
[1]
[1, [2, 3]]
Extend
example_list.append(1)
print(example_list)
example_list.append([2, 3])
print(example_list)
output :
[1]
[1, [2, 3]]
example_list = []
example_list.extend(1)
print(example_list)
example_list.extend([2, 3])
print(example_list)
example_list.extend({'k1' : 'a', 'k2' : 'b'})
print(example_list)
output :
[1]
[1, 2, 3]
[1, 2, 3, 'k1', 'k2']
**Incase of dictionary only keys will be taken, values will be ignored.
Observation : Append will just append the item as a new single item to the list. The item can be an integer or float or list or tuple or set or dictionary where as extend will extend the current list with the elements in the supplied item.
example_list.extend(1)
print(example_list)
example_list.extend([2, 3])
print(example_list)
example_list.extend({'k1' : 'a', 'k2' : 'b'})
print(example_list)
output :
[1]
[1, 2, 3]
[1, 2, 3, 'k1', 'k2']
**Incase of dictionary only keys will be taken, values will be ignored.
Insert
example_list = [1, 2, 3]
example_list.insert(2, 'insertedValue') #2 is index at which item to be inserted
print(example_list)
output :
[1, 2, 'insertedValue', 3]
**If the index is not in range, the element will be inserted at the end of the list.
example_list.insert(2, 'insertedValue') #2 is index at which item to be inserted
print(example_list)
output :
[1, 2, 'insertedValue', 3]
**If the index is not in range, the element will be inserted at the end of the list.
How to delete or remove an item from Python lists
Python list has remove, del and pop functions to delete an item from a list.Remove
example_list = ['a', 'b', 'c', 'a']
print(example_list)
example_list.remove('a')
print(example_list)
output :
['a', 'b', 'c', 'a']
['b', 'c', 'a']
**removes the first occurence of the given item. If the item is not found Python will throw an error.
Del
print(example_list)
example_list.remove('a')
print(example_list)
output :
['a', 'b', 'c', 'a']
['b', 'c', 'a']
**removes the first occurence of the given item. If the item is not found Python will throw an error.
example_list = ['a', 'b', 'c', 'a']
print(example_list)
del example_list[1]
print(example_list)
output :
['a', 'b', 'c', 'a']
['a', 'c', 'a']
**del function uses index to delete the item
pop
print(example_list)
del example_list[1]
print(example_list)
output :
['a', 'b', 'c', 'a']
['a', 'c', 'a']
**del function uses index to delete the item
example_list = ['a', 'b', 'c', 'a']
print(example_list)
example_list.pop()
print(example_list)
example_list.pop(0)
print(example_list)
output :
['a', 'b', 'c', 'a']
['a', 'b', 'c']
['b', 'c']
**pop function deletes the last item and returns it if no index is specified
print(example_list)
example_list.pop()
print(example_list)
example_list.pop(0)
print(example_list)
output :
['a', 'b', 'c', 'a']
['a', 'b', 'c']
['b', 'c']
**pop function deletes the last item and returns it if no index is specified
List slicing
List slicing is nothing but getting another list from the existing list as required. With out any delay lets get into different ways of slicing.
Syntax : list[startIndex:endIndex:stepSize]
**endIndex is always excluded. stepSize is optional
**endIndex is always excluded. stepSize is optional
l = [10, 20, 30, 40, 50, 60, 70, 80] # The list has 8 elements index starts from 0 to 7 new_list = l[0:7] print(new_list) # new_list will be [10, 20, 30, 40, 50, 60, 70] because endIndex 7 is excluded. new_list = l[0:] print(new_list) #If we dont specify the endIndex, python will fetch the last item as well. new_list = l[:] # If you dont specify the startIndex, endIndex python will fetch all the items.
l = [10, 20, 30, 40, 50, 60, 70, 80] Get given range of items new_list = l[0:5] # [10, 20, 30, 40, 50] To get all the elements of a list new_list = l[0:] # [10, 20, 30, 40, 50, 60, 70, 80] new_list = l[:] # [10, 20, 30, 40, 50, 60, 70, 80] new_list = l[::] # [10, 20, 30, 40, 50, 60, 70, 80] To get the list items in reverse order new_list = l[::-1] # [80, 70, 60, 50, 40, 30, 20, 10] Lets see the usage of stepSize new_list = l[0::2] # [10, 30, 50, 70]
Comments
Post a Comment